Bluetooth Low Energy (BLE) technology has revolutionized the way we interact with devices wirelessly, enabling a wide range of applications such as fitness trackers, smartwatches, and IoT devices. To demonstrate the seamless process of connecting to a BLE device and reading its characteristics, this tutorial will guide you through the steps of using the BleuIO JavaScript library. BleuIO simplifies the process of communicating with BLE devices and extracting valuable information from them.
Prerequisites
Before diving into the tutorial, make sure you have the following prerequisites:
- Basic understanding of JavaScript.
- Node.js installed on your system.
- BleuIO Bluetooth Low Energy USB dongle.
- Air quality monitoring BLE device HibouAir (for demonstration purposes).
Step 1: Set Up the Project
- Create a new directory for your project and navigate to it using the terminal.
- Install BleuIO javascript library using
npm i bleuio
- Create an index.html page that contains buttons to connect to the dongle, connecting and reading characteristics value. We will also have a js script linked at the bottom of the page. Here is the full page content
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>Connect and Service data from BLE devices</title>
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.1/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-4bw+/aepP/YC94hEpVNVgiZdgIC5+VKNBQNGCHeKRQN+PtmoHDEXuppvnDJzQIu9"
crossorigin="anonymous"
/>
<style>
#terminal {
background-color: black;
color: white;
font-size: medium;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
padding: 20px;
margin: 20px 0;
}
</style>
</head>
<body>
<div class="container my-3">
<img src="https://www.bleuio.com/images/logo.png" alt="" />
<h1 class="my-4">Example script to connect and read Characteristics</h1>
<button class="btn btn-warning" id="connect">Connect</button>
<button class="btn btn-primary" id="info">Connection Information</button>
<button class="btn btn-success" id="ReadService">
Connect & Read Service Data
</button>
<h3><div id="readResponse"></div></h3>
<div id="terminal"></div>
</div>
<script type="module" src="./script.js"></script>
</body>
</html>
- Create a js page that contains the logic for connecting to dongle and then connect to Air quality monitoring BLE device HibouAir. After connecting to the device we try to read device manufacturing company name by reading characteristics.
following is the script file.
import * as my_dongle from 'bleuio';
document.getElementById('connect').addEventListener('click', function () {
my_dongle.at_connect();
});
document.getElementById('info').addEventListener('click', function () {
my_dongle.ati().then((x) => {
document.getElementById('terminal').innerHTML += x.join('<br>');
});
});
let deviceToConnect = '[1]D1:53:C9:A9:8C:D2';
document.getElementById('ReadService').addEventListener('click', function () {
my_dongle.at_dual().then(() => {
my_dongle.at_gapconnect(deviceToConnect).then((x) => {
setTimeout(() => {
document.getElementById('terminal').innerHTML += x.join('<br>');
my_dongle.at_gattcread('0011').then((x) => {
document.getElementById('terminal').innerHTML += x.join('<br>');
document.getElementById('readResponse').innerHTML = x[x.length - 1];
my_dongle.at_gapdisconnectall();
});
}, 500);
});
});
});
As you can notice on the script we have a variable called device to connect. This is the mac address of the HibouAir BLE device that we are trying to connect. You can replace this mac address to your own device. After the connection we print out the response in a terminal.
on the terminal we can see available service and characteristics. In this script we are trying to read device manufacturer name which is stored at 0011 handle. Therefore we pass the value as at_gattcread function. You can read more about the AT commands at BleuIO getting started guide.
To run this script, we need a web bundler like parcel js.
Run the script with
npx parcel index.html
Example output
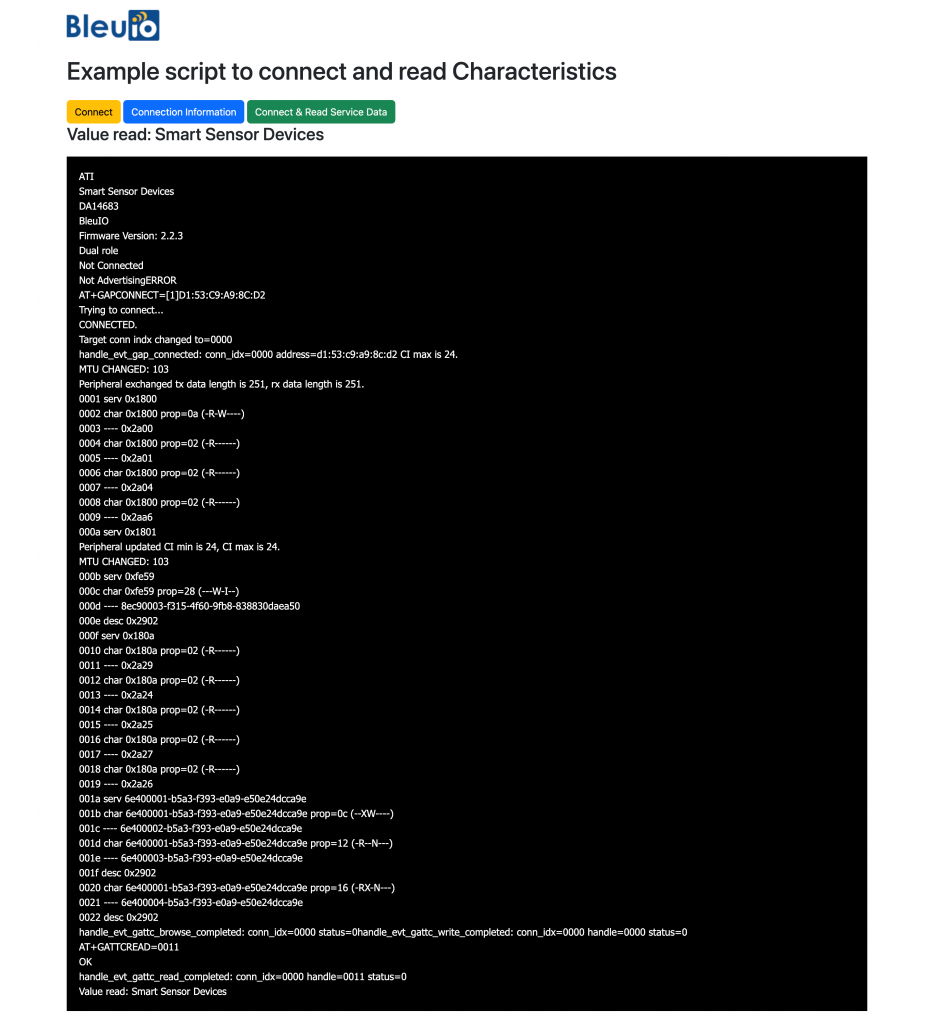
In this tutorial, we’ve successfully demonstrated how to connect to a BLE device using the BleuIO dongle and JavaScript. By leveraging the BleuIO’s Javascript library, we streamlined the process of establishing a connection, reading device characteristics, and displaying the information on a web page. BLE technology has vast potential for various applications, and BleuIO makes it easier than ever to interact with BLE devices programmatically. With this tutorial as a foundation, you can explore further and develop your own BLE-powered projects with confidence.