Bluetooth Low Energy (BLE) is a low-power wireless technology used for connecting devices with each other. It is a popular communication method, especially in the era of the Internet of Things. Several devices around the house have a built-in Bluetooth transceiver and most of them provide useful capabilities to automate jobs. For that reason, it is really interesting to create a desktop application using C# that connects to the devices around the house and manages them.
In this example, we are going to create a simple C# windows form application that scans and shows a list of nearby Bluetooth devices.
Let’s start
As a first step let’s create a new project in visual studio and select C# windows form application from the list.

Choose a suitable name for your project.
Once the project is created, we will see a blank form screen where we will add buttons and labels to communicate with BleuIO graphically through the serial port.
We will have buttons that connect and disconnects from the dongle. We create a button called Scan. And a text area will display a list of Bluetooth devices.
The form will look like this
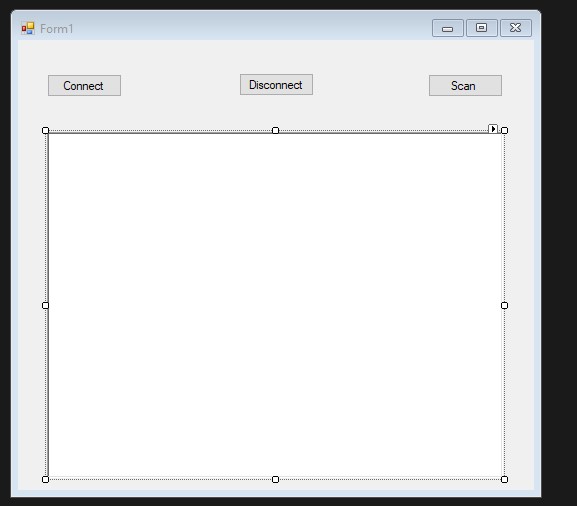
The .cs file associated to this will have the following code.
Source code is available at https://github.com/smart-sensor-devices-ab/c-sharp-scan-bluetooth-device.git
using System;
using System.IO.Ports;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Scan_BLE_devices
{
public partial class Form1 : Form
{
SerialPort mySerialPort = new SerialPort("COM18", 57600, Parity.None, 8, StopBits.One);
public Form1()
{
InitializeComponent();
mySerialPort.DataReceived += new SerialDataReceivedEventHandler(mySerialPort_DataReceived);
mySerialPort.Open();
}
//print response from the dongle
private void mySerialPort_DataReceived(object sender, SerialDataReceivedEventArgs e)
{
SerialPort sp = (SerialPort)sender;
string s = sp.ReadExisting();
output_data.Invoke(new EventHandler(delegate { output_data.Text += s + "\r\n"; }));
//lbl_output.Invoke(this.myDelegate, new Object[] { s });
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void btn_disconnect_Click_1(object sender, EventArgs e)
{
mySerialPort.Close();
Environment.Exit(0);
}
private void button1_Click_1(object sender, EventArgs e)
{
lbl_test.Text = "Connected";
}
private void submit_cmd_Click_1(object sender, EventArgs e)
{
output_data.Text = "";
byte[] bytes = Encoding.UTF8.GetBytes("AT+CENTRAL");
var inputByte = new byte[] { 13 };
bytes = bytes.Concat(inputByte).ToArray();
mySerialPort.Write(bytes, 0, bytes.Length);
System.Threading.Thread.Sleep(1000);
output_data.Text = "";
byte[] bytes2 = Encoding.UTF8.GetBytes("AT+GAPSCAN=3");
var inputByte2 = new byte[] { 13 };
bytes2 = bytes2.Concat(inputByte2).ToArray();
mySerialPort.Write(bytes2, 0, bytes2.Length);
//System.Threading.Thread.Sleep(3000);
}
private void output_data_TextChanged(object sender, EventArgs e)
{
}
}
}
As you can notice I wrote COM18 to connect to serial port because BleuIO device on my computer is connected to COM18.
You can check your COM port from device manager.
Lets run the project and click on connect button.
Once we are connected to BlueIO dongle through serial port, we will be able to scan for nearby Bluetooth device. Clicking on Scan button will show a list of nearby Bluetooth device on the screen.
Output
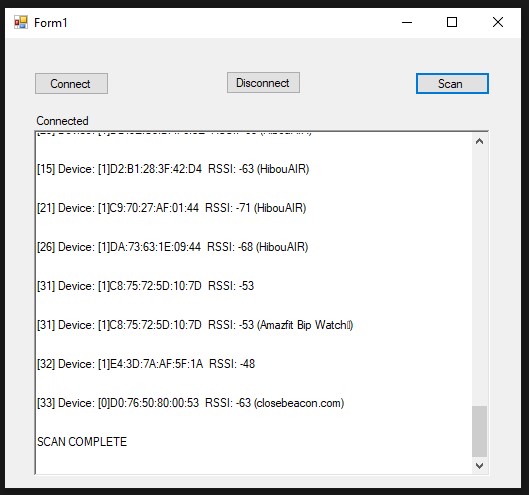