This article takes a deep dive into the capabilities of BleuIO, exploring how it can simplify BLE application testing, especially in complex environments like IoT, health, automotive, and smart home applications. Whether you are a seasoned developer or a newcomer, BleuIO provides a reliable, versatile tool to jumpstart your BLE projects. We’ll also cover an example project, the BLE Connectivity Test Tool, to illustrate BleuIO’s ease of use and the possibilities it opens up for developers.
Example Project: Building a BLE Connectivity Test Tool
To demonstrate the practicality and ease of using BleuIO, let’s look at a sample project: a BLE Connectivity Test Tool. This tool allows developers to test the connection stability of a BLE device, such as the HibouAir air quality monitor, by performing repeated connection and disconnection attempts. This example showcases how easy it is to set up a real-world testing scenario with BleuIO, perfect for those looking to explore connectivity applications.
This BLE Connectivity Test Tool will:
Scan for the HibouAir Device: Use BleuIO to locate the device based on its BLE address.
Initiate Connections: Repeatedly connect and disconnect from the HibouAir device, tracking the success and failure of each attempt.
Log Results: Display a summary at the end, indicating successful connections and any errors encountered.
Code Overview
The Python script below uses the official BleuIO Python library, which simplifies interaction with BLE devices. It uses AT commands to set the dongle in central mode, initiate connections, and disconnect after a set duration.
import time
from datetime import datetime
from bleuio_lib.bleuio_funcs import BleuIO
# Initialize the BleuIO dongle
dongle = BleuIO()
# Device address of HibouAir (replace with your actual address if different)
device_address = "[1]D1:79:29:DB:CB:CC"
# Number of connection attempts
test_count = 5
# Duration to stay connected in seconds
connect_duration = 2
# Delay between connection attempts
delay_between_tests = 5
# Counters for tracking connection results
successful_connections = 0
successful_disconnections = 0
connection_failures = 0
# Register scan callback to handle scanned device responses
def my_scan_callback(scan_input):
print("\n\nScan Result: " + str(scan_input))
# Register event callback to handle connection events with timestamps
def my_evt_callback(evt_input):
cbTime = datetime.now()
currentTime = cbTime.strftime("%H:%M:%S")
print("\n\n[" + str(currentTime) + "] Event: " + str(evt_input))
# Set up the callback functions
dongle.register_evt_cb(my_evt_callback)
dongle.register_scan_cb(my_scan_callback)
# Set the BLE role to central to initiate connections
dongle.at_central()
# Start the connection test
print("Starting BLE Connectivity Test with HibouAir device...\n")
for i in range(test_count):
print(f"\nAttempt {i+1}/{test_count}")
# Connect to the device
connect_response = dongle.at_gapconnect(device_address)
if connect_response.Ack.get("err") == 0: # Check if the connection was successful
print("Connection successful!")
successful_connections += 1
time.sleep(connect_duration) # Stay connected for the specified duration
# Disconnect
disconnect_response = dongle.at_gapdisconnect()
if disconnect_response.Ack.get("err") == 0:
print("Disconnected successfully.")
successful_disconnections += 1
else:
print("Error during disconnect:", disconnect_response.Ack.get("errMsg"))
connection_failures += 1
else:
print("Connection failed:", connect_response.Ack.get("errMsg"))
connection_failures += 1
# Wait before the next attempt
time.sleep(delay_between_tests)
# Display test results summary
print("\nConnectivity Test Summary:")
print(f"Total Connection Attempts: {test_count}")
print(f"Successful Connections: {successful_connections}")
print(f"Successful Disconnections: {successful_disconnections}")
print(f"Failures: {connection_failures}")
This tool’s simplicity shows how BleuIO can be used in the development and testing process, making it a preferred choice for developers who want to avoid BLE’s traditional complexities.
Output
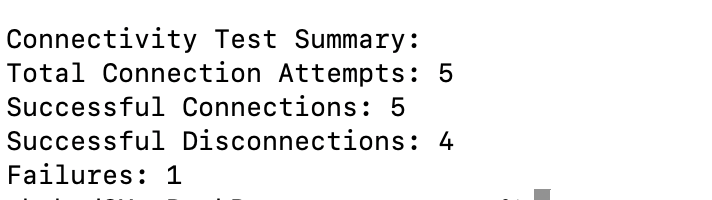
Real-World Applications of BleuIO
IoT Device Testing: From smart home devices to industrial sensors, BleuIO is invaluable for testing the connectivity and data transmission of IoT devices.
Wearable Tech Development: For developers working on BLE-enabled wearables like fitness trackers or health monitors, BleuIO provides a straightforward way to test device connectivity.
Educational and Prototyping Tool: BleuIO’s ease of use makes it an excellent educational tool for introducing BLE concepts to students and rapid prototyping for startups.
Environmental Monitoring: As demonstrated with the HibouAir air quality monitor, BleuIO facilitates connectivity testing in applications involving environmental data, like CO2 monitoring, temperature, and humidity.
Why BleuIO for BLE Development?
Developing BLE applications often requires specialized hardware and software expertise. Traditional BLE development involves understanding intricate details of Bluetooth stacks, profiles, and data protocols. BleuIO alleviates this by offering a straightforward command structure that abstracts the technical details, allowing developers to quickly build and test applications.
In addition, BleuIO’s compact design makes it suitable for portable applications and rapid prototyping. From sensor connectivity to device tracking and monitoring, BleuIO proves to be a versatile asset for a range of BLE applications.
BleuIO offers an all-in-one solution for BLE development, bridging the gap between beginner-friendly usability and powerful functionality. With support for multiple platforms, easy integration with popular programming languages, and straightforward AT command access, BleuIO empowers developers to quickly and efficiently test, prototype, and deploy BLE applications.
By using tools like the BLE Connectivity Test Tool, developers can focus on creating innovative applications without needing to dive deep into the complexities of BLE. BleuIO enables developers to bring their ideas to life, from IoT to healthcare and beyond. Start your BLE development journey with BleuIO today and see the difference in ease and efficiency it brings to your projects.