Using BleuIO for BLE Application Development with C++
April 5, 2024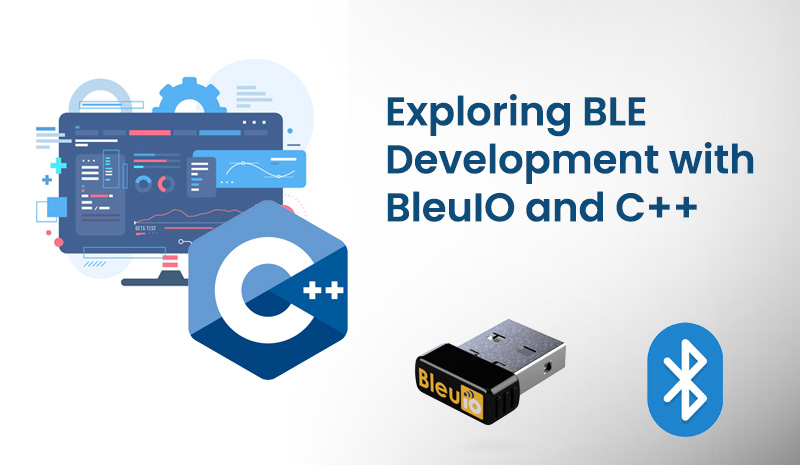
In this tutorial, we will explore how to use BleuIO, a Bluetooth Low Energy (BLE) USB dongle, for developing BLE applications with C++. We’ll demonstrate how to connect to BleuIO via a serial port, send AT commands, and receive responses. Additionally, we’ll discuss the benefits of using BleuIO and provide some use cases for BLE application development with C++.
Introduction to BleuIO
BleuIO is a BLE USB dongle designed to simplify and accelerate the development of BLE applications. It provides a set of AT commands that allow developers to interact with BLE devices and services without having to write extensive code. By leveraging these AT commands, developers can quickly prototype and develop BLE applications with minimal effort.
Prerequisites
Before getting started, ensure you have the following:
- BleuIO BLE USB dongle
- Serial communication library for C++ (e.g.,
<iostream>
,<unistd.h>
,<fcntl.h>
,<termios.h>
) - Basic knowledge of C++ programming
Setting Up the Environment
First, connect the BleuIO dongle to your computer via USB. Then, (for MAC operating system) determine the serial port assigned to the dongle using the ls /dev/cu.*
command in the terminal. Note down the serial port name (e.g., /dev/cu.usbmodem4048FDE52DAF1
) as we’ll need it to establish a serial connection.
Writing the C++ Program
Below is a C++ program that connects to BleuIO, sends an AT command, and retrieves the response.
#include <iostream>
#include <string>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <chrono>
#include <thread>
int main() {
// Open the serial port
const char* portname = "/dev/cu.usbmodem4048FDE52DAF1"; // Change this to match your serial port
int serial_port = open(portname, O_RDWR);
if (serial_port < 0) {
std::cerr << "Error opening serial port" << std::endl;
return 1;
}
// Configure the serial port settings
struct termios tty;
tcgetattr(serial_port, &tty);
cfsetospeed(&tty, B9600); // Set baud rate to 9600
cfsetispeed(&tty, B9600);
tty.c_cflag &= ~PARENB; // No parity
tty.c_cflag &= ~CSTOPB; // 1 stop bit
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8; // 8 bits per byte
tty.c_cflag &= ~CRTSCTS; // Disable hardware flow control
tty.c_cflag |= CREAD | CLOCAL; // Enable reading and ignore control lines
tty.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); // Raw input
tcsetattr(serial_port, TCSANOW, &tty);
// Write data to the serial port to put the dongle in central role
const char* m1 = "AT+CENTRAL\r";
write(serial_port, m1, strlen(m1));
// Wait for 1 second to receive the response
std::this_thread::sleep_for(std::chrono::seconds(1));
// Write data to the serial port to scan for nearby device for three seconds
const char* m2 = "AT+GAPSCAN=3\r";
write(serial_port, m2, strlen(m2));
// Wait for 1 second to receive the response
std::this_thread::sleep_for(std::chrono::seconds(1));
// Read response from the serial port with timeout
char buf[1024];
std::string response;
int nbytes;
fd_set fds;
struct timeval timeout;
FD_ZERO(&fds);
FD_SET(serial_port, &fds);
timeout.tv_sec = 3; // Timeout after 3 second
timeout.tv_usec = 0;
while (select(serial_port + 1, &fds, NULL, NULL, &timeout) > 0) {
nbytes = read(serial_port, buf, sizeof(buf));
if (nbytes < 0) {
std::cerr << "Error reading from serial port" << std::endl;
close(serial_port);
return 1;
}
if (nbytes > 0) {
response.append(buf, nbytes);
}
}
// Display the response
std::cout << "Response: " << response << std::endl;
// Close the serial port
close(serial_port);
return 0;
}
Understanding the Code
- We start by opening the serial port corresponding to BleuIO.
- Next, we configure the serial port settings (baud rate, parity, stop bits, etc.) to match BleuIO’s communication parameters.
- We then send the
AT
+CENTRAL command to BleuIO, which puts the dongle into central role. - After sending the command, we wait for 1 second to receive the response.
- Then we send AT+GAPSCAN=3 to scan for nearby BLE devices for 3 seconds.
- We read the response from the serial port with a timeout of 3 second.
- Finally, we display the response received from BleuIO and close the serial port.
Output
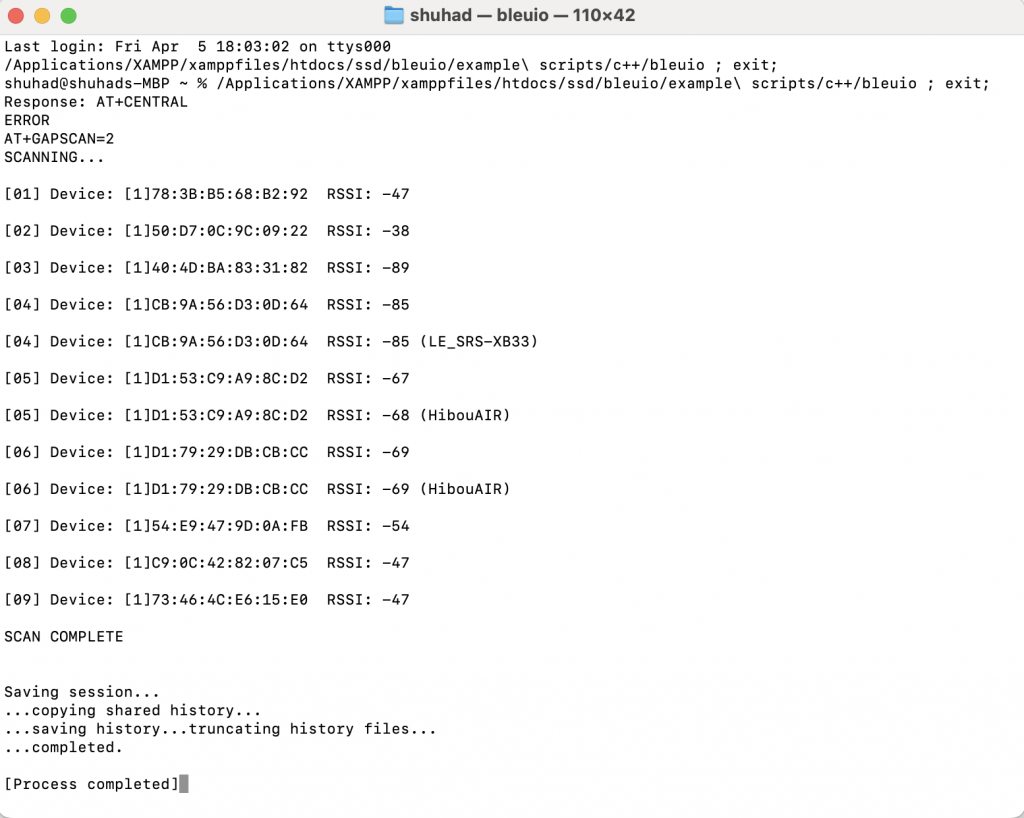
Use Cases
BleuIO, with its AT command interface, presents an excellent starting point for developers looking to delve into Bluetooth Low Energy (BLE) application development with C++ across various domains.
Embedded Systems Development: BleuIO simplifies BLE application development in C++ for embedded systems. Developers can quickly prototype IoT devices, smart sensors, and wearables with BleuIO’s AT command interface, enabling features like remote control and wireless connectivity.
Cross-Platform Mobile Applications: Integrating BLE features into C++ mobile apps becomes seamless with BleuIO. Developers using frameworks like Qt or cross-platform tools can leverage BleuIO’s AT commands for device discovery, data exchange, and configuration across different platforms.
Industrial Automation and Control: C++ developers in industrial settings can enhance control systems and monitoring tools with BLE connectivity using BleuIO. Its AT command support enables communication with BLE-enabled equipment for tasks like remote monitoring and real-time data acquisition.
Educational Projects and Prototyping: BleuIO serves as an accessible platform for learning BLE technology with C++. Students and hobbyists can experiment with Bluetooth communication, protocol implementation, and IoT development, turning ideas into reality with minimal overhead.
In this tutorial, we’ve demonstrated how to use BleuIO for BLE application development with C++. By leveraging BleuIO’s AT commands, developers can streamline the development process and accelerate time-to-market for BLE-enabled projects. With the provided code and insights, you can now begin exploring BLE application development using BleuIO and C++ in your own projects.
For more information about BleuIO and its capabilities, refer to the official documentation and resources.