Developing BLE Applications with BleuIO and Rust
May 10, 2024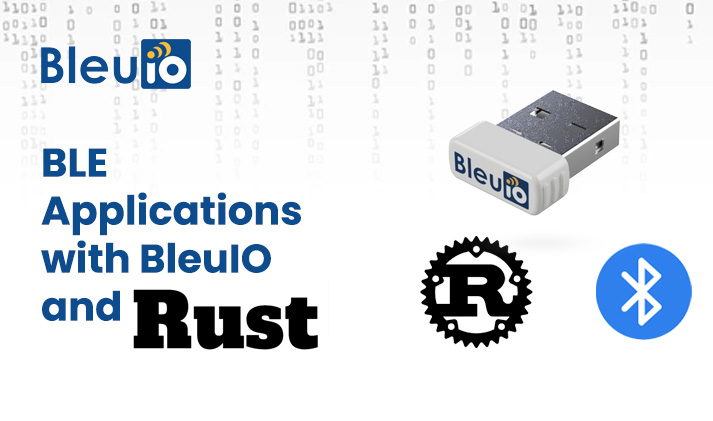
BleuIO is a versatile Bluetooth Low Energy (BLE) USB dongle designed to simplify the development of BLE applications. With its support for AT commands and seamless integration with Rust programming language, BleuIO offers developers a straightforward and efficient way to create BLE applications. In this tutorial, we will explore how to use BleuIO and Rust to develop BLE applications easily.
About Rust Programming Language:
Rust is a modern, systems programming language that focuses on safety, performance, and concurrency. Developed by Mozilla, Rust has gained popularity for its unique features and advantages, making it an excellent choice for various application domains, including system programming, web development, and embedded systems.
Prerequisites:
- Basic knowledge of Rust programming language
- BleuIO Bleutooth Low Energy USB dongle
- Computer with Rust compiler and serial communication capabilities
Setting Up BleuIO:
- Connect BleuIO Dongle:
- Connect the BleuIO dongle to an available USB port on your computer.
- Identify Serial Port:
- Identify the serial port associated with BleuIO. For example, On macOS and Linux, it may look like
/dev/cu.usbmodem4048FDE52DAF1
. On windows it looks like COM6
- Identify the serial port associated with BleuIO. For example, On macOS and Linux, it may look like
Installing Rust and Cargo:
- Windows:
- Download and install the Rust compiler (including Cargo) from the official website: Rustup.
- Follow the installation instructions provided on the website.
- Mac/Linux:
- Open a terminal and run the following command to install Rust and Cargo:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Follow the on-screen instructions to complete the installation.
Creating a Cargo Project:
- Open Terminal/Command Prompt:
- Windows: Open Command Prompt or PowerShell.
- Mac/Linux: Open Terminal.
- Navigate to Project Directory:
cd /path/to/projects
- Create a New Cargo Project:
cargo new BleuIO
This will create a new directory named “BleuIO” containing the project files.
- Navigate into the Project Directory:
cd BleuIO
Writing BLE Application Code:
- Open
src/main.rs
in Your Code Editor:- Replace the default Rust code with the BLE application code. Make sure to replace the port_name with your connected BleuIO port.
- Implement BLE Application Logic:
- Write Rust code to interact with BleuIO using AT commands.
use std::io::{self, Write};
use std::thread::sleep;
use std::time::Duration;
use serialport;
fn main() -> io::Result<()> {
// Open the serial port
let port_name = "/dev/cu.usbmodem4048FDE52DAF1";
let mut port = serialport::new(port_name, 9600)
.timeout(Duration::from_secs(5)) // Adjust timeout value here (5 seconds in this example)
.open()
.map_err(|e| io::Error::new(io::ErrorKind::Other, e))?;
// Write "AT+CENTRAL" to set the BleuIO dongle to centrla role
let data_central = b"AT+CENTRAL\r\n";
port.write_all(data_central).map_err(|e| io::Error::new(io::ErrorKind::Other, e))?;
// Wait for 500 milliseconds
sleep(Duration::from_millis(5));
// Write "AT+GAPSCAN=3" to scan for nearby BLE devices for 3 seconds
let data_gapscan = b"AT+GAPSCAN=3\r\n";
port.write_all(data_gapscan).map_err(|e| io::Error::new(io::ErrorKind::Other, e))?;
// Read response from the BleuIO dongle until no more data is available
let mut response = String::new();
loop {
let mut buffer: [u8; 128] = [0; 128];
let bytes_read = port.read(&mut buffer).map_err(|e| io::Error::new(io::ErrorKind::Other, e))?;
// Check if no more data is available
if bytes_read == 0 {
break;
}
// Convert bytes to string and append to the response
let chunk = String::from_utf8_lossy(&buffer[..bytes_read]);
response.push_str(&chunk);
// Print the current chunk of response
print!("{}", chunk);
}
// Drop the port to close it
drop(port);
Ok(())
}
Building and Running the Project:
- Build the Project:
cargo build
- Run the Project:
cargo run
Output
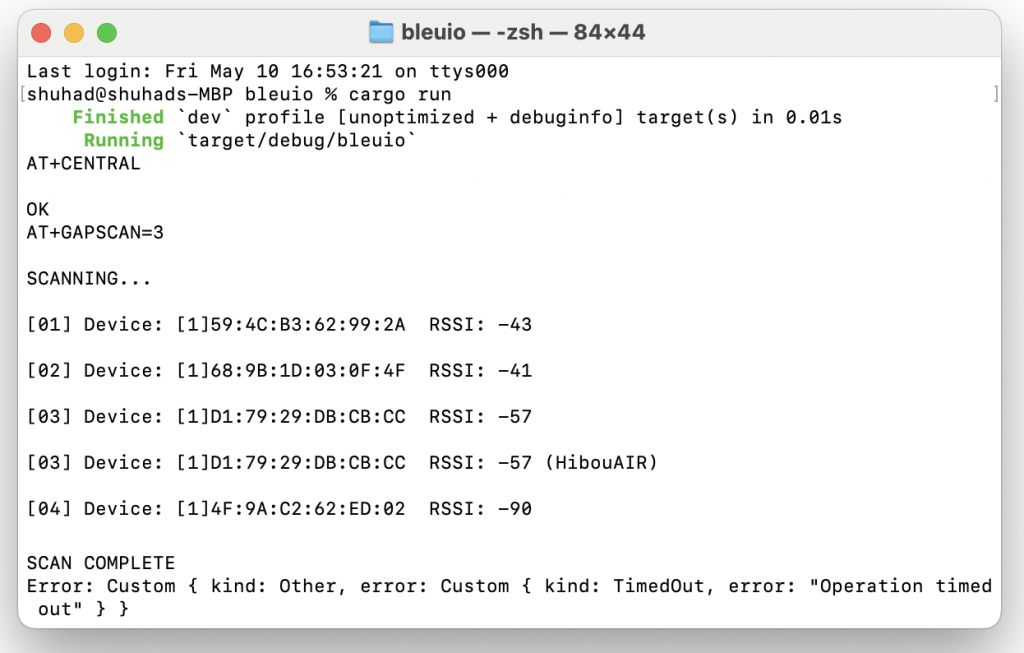
In this tutorial, we’ve demonstrated how to develop a simple BLE applications using BleuIO and Rust that puts the BleuIO in central role and scans for nearby BLE devices for 3 seconds. Finally shows the list on the screen. By using BleuIO’s support for AT commands and Rust’s simplicity, developers can create BLE applications effortlessly. Start exploring the possibilities with BleuIO and Rust today!